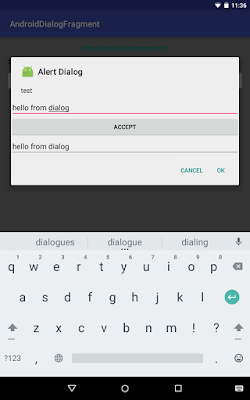
This example modify from last example of "Create DialogFragment with AlertDialog.Builder", to implement interactive DialogFragment. Something sent from main activity to DialogFragment, and something editable in DialogFragment, and something sent from DialogFragment back to main activity.
MainActivity.java
package com.blogspot.android_er.androiddialogfragment;
import android.app.AlertDialog;
import android.app.Dialog;
import android.app.DialogFragment;
import android.app.Fragment;
import android.app.FragmentTransaction;
import android.content.DialogInterface;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.LinearLayout;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
EditText inputTextField;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
inputTextField = (EditText)findViewById(R.id.inputtext);
Button btnOpen = (Button)findViewById(R.id.opendialog);
btnOpen.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
showDialog();
}
});
}
void showDialog() {
FragmentTransaction ft = getFragmentManager().beginTransaction();
Fragment prev = getFragmentManager().findFragmentByTag("dialog");
if (prev != null) {
ft.remove(prev);
}
ft.addToBackStack(null);
String inputText = inputTextField.getText().toString();
DialogFragment newFragment = MyDialogFragment.newInstance(inputText, inputTextField);
newFragment.show(ft, "dialog");
}
public static class MyDialogFragment extends DialogFragment {
String mText;
static EditText backTextField;
static MyDialogFragment newInstance(String text, EditText editText) {
backTextField = editText;
MyDialogFragment f = new MyDialogFragment();
Bundle args = new Bundle();
args.putString("text", text);
f.setArguments(args);
return f;
}
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
mText = getArguments().getString("text");
//create custom LinearLayout programmatically
LinearLayout layout = new LinearLayout(getActivity());
layout.setLayoutParams(new LinearLayout.LayoutParams(
LinearLayout.LayoutParams.MATCH_PARENT,
LinearLayout.LayoutParams.WRAP_CONTENT));
layout.setOrientation(LinearLayout.VERTICAL);
final EditText editField = new EditText(getActivity());
editField.setHint("type something");
Button btnAccept = new Button(getActivity());
btnAccept.setText("Accept");
final EditText textField = new EditText(getActivity());
btnAccept.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
textField.setText(editField.getText().toString());
}
});
layout.addView(editField);
layout.addView(btnAccept);
layout.addView(textField);
return new AlertDialog.Builder(getActivity())
.setIcon(R.mipmap.ic_launcher)
.setTitle("Alert Dialog")
.setMessage(mText)
.setPositiveButton("OK",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int whichButton) {
Toast.makeText(getActivity(),
textField.getText(),
Toast.LENGTH_LONG).show();
backTextField.setText(textField.getText());
}
}
)
.setNegativeButton("Cancel", null)
.setView(layout)
.create();
}
}
}
layout/activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
android_layout_width="match_parent"
android_layout_height="match_parent"
android_padding="16dp"
android_orientation="vertical"
tools_context=".MainActivity"
android_background="#808080">
<TextView
android_layout_width="wrap_content"
android_layout_height="wrap_content"
android_layout_gravity="center_horizontal"
android_autoLink="web"
android_text="http://android-er.blogspot.com/"
android_textStyle="bold" />
<EditText
android_id="@+id/inputtext"
android_layout_width="match_parent"
android_layout_height="wrap_content"
android_hint="Type something"/>
<Button
android_id="@+id/opendialog"
android_layout_width="match_parent"
android_layout_height="wrap_content"
android_text="Open DialogFragment"
android_textAllCaps="false"/>
</LinearLayout>